Custom DateTimeJsonConverter in .NET for Enhanced JSON Serialization
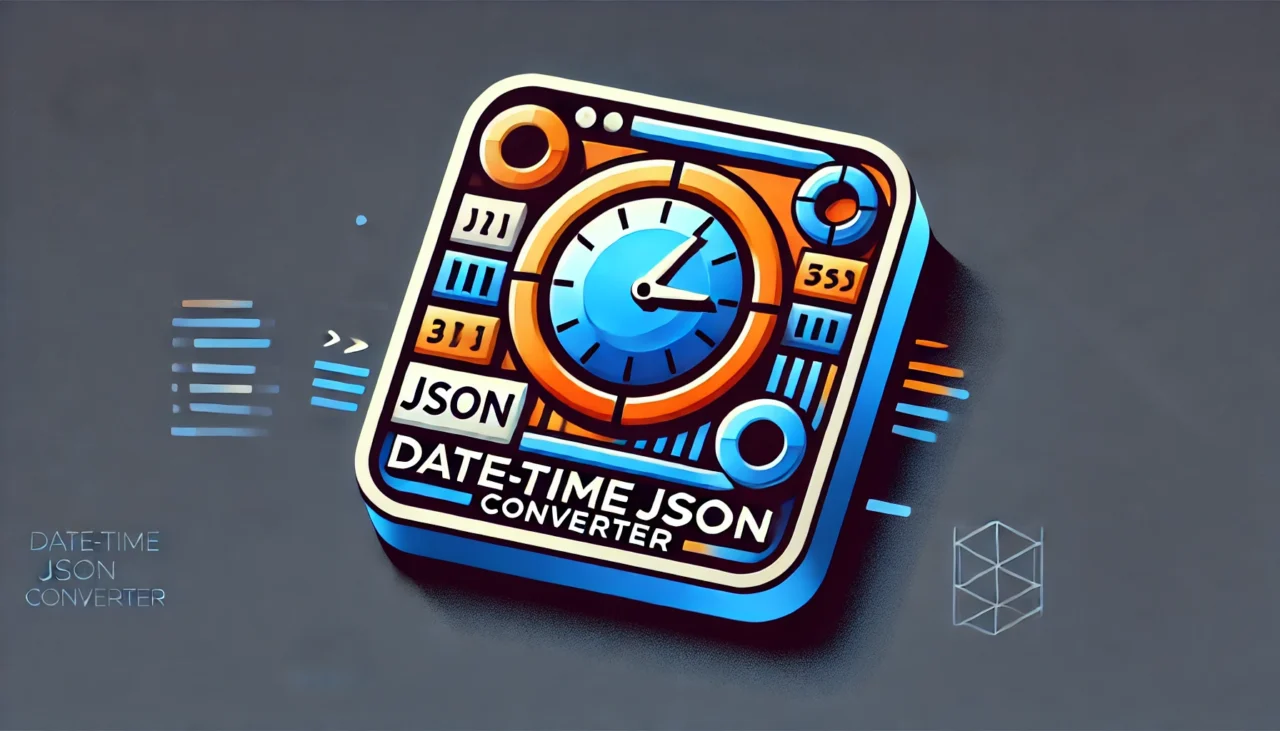
When working with JSON serialization in .NET, one of the common challenges developers face is formatting DateTime
and DateTimeOffset
properties. By default, the .NET JsonSerializer
doesn’t provide a built-in way to specify custom formats for individual properties. This limitation can lead to workarounds that are cumbersome and error-prone, such as applying global settings or manual formatting during serialization. However, with the DateTimeJsonConverter
available in the NuvTools.Common library, this gap can be effectively addressed.
Installing the NuvTools.Common Library
To use the DateTimeJsonConverter
, first install the NuvTools.Common library via NuGet:
NuGet\Install-Package NuvTools.Common
The source code for this library is open-source and can be found on GitHub: https://github.com/nuvtools/nuvtools-common
The specific implementation of the DateTimeJsonConverter
is located under: Serialization/Json/Converters
Overview of the DateTimeJsonConverter
The DateTimeJsonConverter
is a custom JSON converter that allows you to specify a format for serializing and deserializing DateTime
and DateTimeOffset
properties. This feature is implemented as an attribute, making it incredibly straightforward to apply to individual properties.
Here’s how the DateTimeJsonConverter
works:
- It decorates the property with the desired date format.
- It integrates seamlessly with the
JsonSerializer
. - It eliminates the need for global converters or additional logic for individual formatting.
How to Use the DateTimeJsonConverter
1. Decorate Properties with the Attribute
To apply a specific format to a DateTime
property, use the DateTimeJsonConverter
attribute:
using NuvTools.Common.Serialization.Json.Converters; public class SampleModel { [DateTimeJsonConverter("dd/MM/yyyy")] public DateTime CustomFormattedDate { get; set; } public string Description { get; set; } }
2. Serialize and Deserialize JSON
With the property decorated, serialization and deserialization automatically respect the specified format:
var model = new SampleModel { CustomFormattedDate = DateTime.Now, Description = "This is a sample model." }; var options = new JsonSerializerOptions { WriteIndented = true }; var json = JsonSerializer.Serialize(model, options); Console.WriteLine(json);
Output:
{ "CustomFormattedDate": "11/01/2025", "Description": "This is a sample model." }
3. Deserialization
When deserializing, the converter automatically parses the incoming date string using the specified format:
var json = "{ \"CustomFormattedDate\": \"11/01/2025\", \"Description\": \"This is a sample model.\" }"; var deserializedModel = JsonSerializer.Deserialize<SampleModel>(json); Console.WriteLine(deserializedModel.CustomFormattedDate); // Outputs: 01/11/2025 00:00:00
Advantages of Using the DateTimeJsonConverter
- Granular Control:
- The
DateTimeJsonConverter
allows you to define date formats on a per-property basis. This eliminates the need for global settings that may affect unrelated parts of your application.
- The
- Ease of Use:
- Applying the converter is as simple as adding an attribute to the property. There is no need for complex serialization logic.
- Integration:
- Works seamlessly with
System.Text.Json
without requiring additional configuration.
- Works seamlessly with
- Maintainability:
- By isolating formatting logic to specific properties, it becomes easier to manage changes over time without unintended side effects.
- Open Source:
- The library is actively maintained and available on GitHub, making it a reliable choice for production applications.
Why This Feature Isn’t Available Out-of-the-Box
While System.Text.Json
provides a powerful and efficient JSON serialization engine, it lacks support for property-specific formatting out of the box. Custom converters can be applied globally, but there is no native mechanism to bind them to individual properties.
The DateTimeJsonConverter
from the NuvTools.Common library fills this gap by leveraging the extensibility of System.Text.Json
through attributes. It abstracts away the complexity of implementing a custom converter for each use case, offering a simple yet robust solution.
Conclusion
The DateTimeJsonConverter
from NuvTools.Common is a game-changer for developers who need precise control over DateTime
and DateTimeOffset
serialization. By providing an attribute-based approach, it offers a level of flexibility and simplicity that is unmatched by native .NET capabilities.
If you’re looking to enhance your JSON serialization workflow, consider incorporating the DateTimeJsonConverter
into your projects. With its open-source nature and ease of use, it’s a must-have tool for modern .NET applications.