500x Faster: Avoid Queries in Loops with C# Entity Framework for Peak Performance
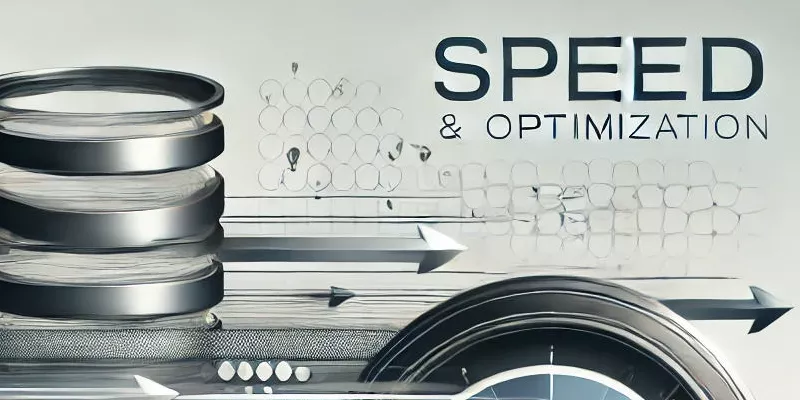
Executing database queries inside a loop is a common mistake that leads to significant performance issues, mainly in high-scale systems. Each iteration of the loop triggers a separate database query, resulting in:
- Increased latency due to multiple network round trips.
- Strained database resources from processing numerous queries.
- High operational costs for cloud-hosted databases with usage-based pricing.
For example, consider the following code in C# utilizing Entity Framework Core:
foreach (var userId in userIds) { var user = await _dbContext.Users.FirstOrDefaultAsync(u => u.Id == userId); if (user != null) Console.WriteLine(user.Name); }
This approach executes one query for each user ID in the list. If the list contains 1,000 user IDs, this results in 1,000 separate database calls, significantly impacting performance.
Performance Comparison: With and Without Loop Query
To illustrate the performance difference, consider the following scenario:
- Dataset: 1,000 user IDs
- Network latency per query: 10ms
- Database query processing time: 5ms per query
Queries Inside a Loop
- Number of Queries: 1,000
- Time per Query: 10 ms (network)+5 ms (processing)=15 ms10 \, \text{ms (network)} + 5 \, \text{ms (processing)} = 15 \, \text{ms}10ms (network)+5ms (processing)=15ms
- Total Time:
1,000×15 ms=15,000 ms (15 seconds)1,000 \times 15 \, \text{ms} = 15,000 \, \text{ms (15 seconds)}1,000×15ms=15,000ms (15 seconds)
Optimized Batch Query
Instead of querying within the loop, retrieve all required data in one query:
var users = await _dbContext.Users .Where(u => userIds.Contains(u.Id)) .ToListAsync(); foreach (var user in users) Console.WriteLine(user.Name);
- Number of Queries: 1
- Time for Query: 10 ms (network)+20 ms (batch processing)=30 ms10 \, \text{ms (network)} + 20 \, \text{ms (batch processing)} = 30 \, \text{ms}10ms (network)+20ms (batch processing)=30ms
- Total Time: 30ms
Performance Gain:
The optimized approach is approximately 500 TIMES FASTER than the query-inside-loop approach.
Advantages of Avoiding Queries in Loops
- Reduced Latency: Consolidating queries minimizes network round trips.
- Improved Performance: Operations complete significantly faster.
- Lower Database Load: One query replaces hundreds or thousands of individual queries.
- Cost Efficiency: Reduced resource usage lowers operational costs.
- Enhanced Scalability: Applications handle larger datasets and higher user concurrency effectively.
Conclusion
Avoiding queries inside loops is a simple yet powerful optimization technique that can significantly improve the performance and scalability of your application. By fetching data in bulk and processing it in memory, you reduce database load, minimize latency, and write cleaner, more maintainable code. In high-scale systems, these optimizations are not just best practices—they are essential for delivering a fast, reliable, and cost-effective solution. By adopting this approach in C# Entity Framework, you can ensure your application is well-equipped to handle growing datasets and user demands without compromising performance.